유니티(Unity) MapBox를 이용한 지도 구축
ar 프로젝트 진행중에 현재 지역의 지도를 구현해야 할 일이 생겨 시도 해보기로 했습니다! 구글맵 api도 있지만 먼저 map box부터 먼저 시도해보겠습니다. 가장 먼저 맵 박스 회원가입을 해줍시다!
ljhyunstory.tistory.com
저번글에 이어서 이번엔 구글 맵 api 를 사용해서 지도를 구현해보도록 하겠습니다!
가장 먼저 구글 api키가 필요합니다!
Google Maps Platform - Location and Mapping Solutions
Create real world and real time experiences for your customers with dynamic maps, routes & places APIs from Google Maps Platform’s location solutions.
mapsplatform.google.com
위의 사이트에서 로그인!
바로 겟 스타트 하시면 됩니다
카드결제 정보까지 등록해서... 일정 사용량 넘어가면 결제되는줄 알았지만...
그렇진 않은것 같습니다!
일단 이렇게 등록을 다하시면 key도 발급 받으실수 있으실껍니다!
다 하시게 되면 아래처럼 api키를 획득하게 됩니다!
그리고 api를 눌러보면 많은 api들이 있습니다. 여기서 제가 사용할 api는 maps static api로 정적 맵을 보여주는 api !
없다면
Google Cloud console
console.cloud.google.com
이제 위의 과정을 다 끝냈다면 코드 부분으로 가봅시다.
Raw 이미지 부터 하나 만들어 주고
Mapmanager을 만들어 줍시다.
저는 현재 위치를 받아 그위치에 대한 api 지도를 받아오는 방식으로 구성 했습니다.
using UnityEngine.Networking;
using UnityEngine.UI;
using UnityEngine;
using System.Collections;
using UnityEngine.Timeline;
public class MapManager : MonoBehaviour
{
public RawImage mapRawImage;
[Header("Map SET")]
public string strBaseURL = "";
public int zoom = 14;
public int mapWidth;
public int mapHeight;
public string strAPIKey = "";
public GPSlocation GPSlocation;
private double latitude = 0;
private double longitude = 0;
private double save_latitude = 0;
private double save_longitude = 0;
// Start is called before the first frame update
void Start()
{
mapRawImage = GetComponent<RawImage>();
StartCoroutine(WaitForSecond());
}
private void Update()
{
latitude = GPSlocation.latitude;
longitude = GPSlocation.longitude;
//print("location" + latitude + " " + longitude);
}
IEnumerator WaitForSecond()
{
while (true)
{
if (save_latitude != latitude || save_longitude != longitude)
{
save_latitude = latitude;
save_longitude = longitude;
StartCoroutine(LoadMap());
}
print("3초");
yield return new WaitForSeconds(3f);
}
yield return new WaitForSeconds(1f);
}
}
여기까지가 현재 경도 위도 상 좌표를 받아와 3초마다 LoadMap를 실행 시켜주는 부분입니다.
다음은 가장 중요한 LoadMap부분!
IEnumerator LoadMap()
{
string url = strBaseURL + "center=" + latitude + "," + longitude +
"&zoom=" + zoom.ToString() +
"&size=" + mapWidth.ToString() + "x" + mapHeight.ToString()
+ "&key=" + strAPIKey;
Debug.Log("URL : " + url);
url = UnityWebRequest.UnEscapeURL(url);
UnityWebRequest req = UnityWebRequestTexture.GetTexture(url);
yield return req.SendWebRequest(); //req값 반환!
mapRawImage.texture = DownloadHandlerTexture.GetContent(req); // 맵 >> 이미지에 적용
}
구글 url을 직접 만들어서 들고 오는 방식을 사용했습니다.
그리고 저의 위치를 받아오기위해서 만든
GPSlocation 스크립을 만들어 주었습니다.
저번에 쓴글을 보고 만들어 주었습니다!
유니티(Unity) 모바일 GPS 위도 경도 받아오기
최근 새로운 팀 프로젝트를 시작하게되어 구현하다 보니 모바일에서 GPS 정보를 받아오는 기능이 필요하여 정리해보았습니다. 정보를 불러오는것 까지는 그렇게 어렵지 않지만 사용법에 대해서
ljhyunstory.tistory.com
using System.Collections;
using System.Collections.Generic;
using System.Runtime.InteropServices;
using UnityEngine.UI;
using UnityEngine;
using UnityEngine.Android;
using System;
using static UnityEngine.EventSystems.EventTrigger;
using Unity.VisualScripting;
using TMPro;
using UnityEngine.XR.ARFoundation;
public class GPSlocation : MonoBehaviour
{
[HideInInspector]
public double latitude=0;
[HideInInspector]
public double longitude=0;
[HideInInspector]
public float delay;
[HideInInspector]
public float maxtime = 5.0f;
[HideInInspector]
public bool receiveGPS = false;
double detailed_num = 1.0;
private void Start()
{
StartCoroutine(Gps_man());
Input.compass.enabled = true;
}
void Update()
{
}
IEnumerator Gps_man()
{
if (!Permission.HasUserAuthorizedPermission(Permission.FineLocation)) // 권한 요청하기 // GPS 요청
{
Permission.RequestUserPermission(Permission.FineLocation);
while (!Permission.HasUserAuthorizedPermission(Permission.FineLocation))
{
yield return null;
}
}
Input.location.Start(0.1f, 0.1f); ;
while (Input.location.status == LocationServiceStatus.Initializing && delay < maxtime)
{
yield return new WaitForSeconds(1);
delay++;
}
receiveGPS = true;
while (receiveGPS)
{
latitude = (double)Input.location.lastData.latitude;
longitude = (double)Input.location.lastData.longitude;
yield return new WaitForSeconds(0.2f);
}
}
}
(쓸데없는 부분들이 있을지도..)
이렇게 현재 저의 위치를 계속 최신화 시켜주며 그위치를 가지고 3초마다 새로운 구글 지도를 만들어 열어주었습니다!
잘 갱신 되는것 같습니다~
참고한 블로그!
[Unity] Static Google Map API 활용기
구글 API 를 사용하려면 구글 클라우드 플랫폼으로 접속하여야 한다. 구글 Maps api는 유료 서비스이며 200달러의 크레딧을 무료로 제공한다. 200달러를 초과할 시 비용이 청구 될 수 있다. https://mapsp
tkablog.tistory.com
틀린점이 있다면 댓 달아주세요!
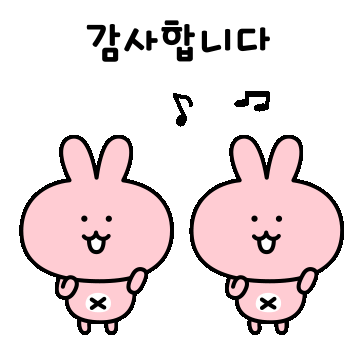
'유니티 최고 > 유니티 구현' 카테고리의 다른 글
유니티(Unity) MapBox를 이용한 지도 구축(2)(Map sty) (1) | 2023.04.12 |
---|---|
유니티(Unity) 웹뷰 사용해보기 (2가지)-Gree &Game Package Manager (1) | 2023.04.10 |
유니티(Unity) MapBox를 이용한 지도 구축 (2) | 2023.03.30 |
유니티(Unity) 모바일 GPS 위도 경도 받아오기 (0) | 2023.03.13 |
유니티(Unity) Photon을 이용한 멀티 플레이 구현하기 (간단) (1) | 2023.02.03 |
댓글